I was making a game where you get money for clicking on a button. Here’s a part of it:
var money = 0; var currentMoney = document.getElementById("currentMoney"); function addOne() { money++; currentMoney.innerHTML = money; }
<button onclick="addOne();">+1$</button> <p>Money: <b id="currentMoney">0</b>$</p>
Later i was looking in the Internet and i found a website called Cookie Clicker and i saw this animation that when you click the cookie, a +1 is going up and fades away (It is not so important that the number goes up where the mouse is for my example):
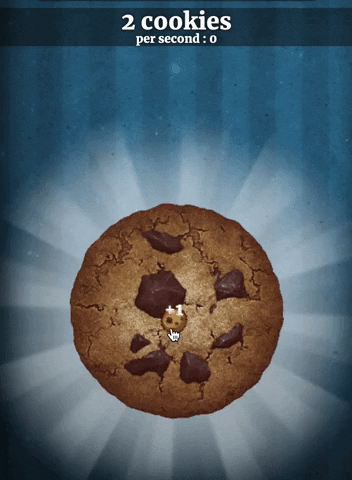
I tried making this animation but i don’t know how i can make it go up:
var money = 0; var currentMoney = document.getElementById("currentMoney"); function addOne() { money++; currentMoney.innerHTML = money; var moneyAnimation = document.createElement("p"); moneyAnimation.innerHTML = "+1"; document.getElementById("moneyAnimation").appendChild(moneyAnimation); }
* { margin: 0; padding: 0; } #moneyAnimation { height: 500px; border: 1px solid; width: 200px; float: right; }
<button onclick="addOne();">+1$</button> <p>Money: <b id="currentMoney">0</b>$</p> <div id="moneyAnimation"></div>
Thanks in advance, have a nice day! 🙂
Advertisement
Answer
I would recommend using CSS animation like below. You can read more about keyframe animation here and here
var money = 0; var currentMoney = document.getElementById("currentMoney"); function addOne() { money++; currentMoney.innerHTML = money; var moneyAnimation = document.createElement("p"); moneyAnimation.innerHTML = "+1"; document.getElementById("moneyAnimation").appendChild(moneyAnimation); moneyAnimation.classList.add("moneyAnimation"); // Add the class that animates }
* { margin: 0; padding: 0; } #moneyAnimation { height: 500px; border: 1px solid; width: 200px; float: right; position: relative; /* Added */ } @keyframes moneyAnimation { 0% { opacity: 1; top: 0; } 100% { opacity: 0; top: -50px; } } .moneyAnimation { animation: moneyAnimation 1s forwards; position: absolute; }
<button onclick="addOne();">+1$</button> <p>Money: <b id="currentMoney">0</b>$</p> <div id="moneyAnimation"></div>